Concurrency Control — Multi-User Environment
In a multi-user environment, when multiple requests are trying to update the same data concurrently, a race condition can occur. Let’s break down the scenario using the provided code as an example
Read Operation
- Two requests simultaneously try to read the current count value from the database.
- Both requests execute the following code:
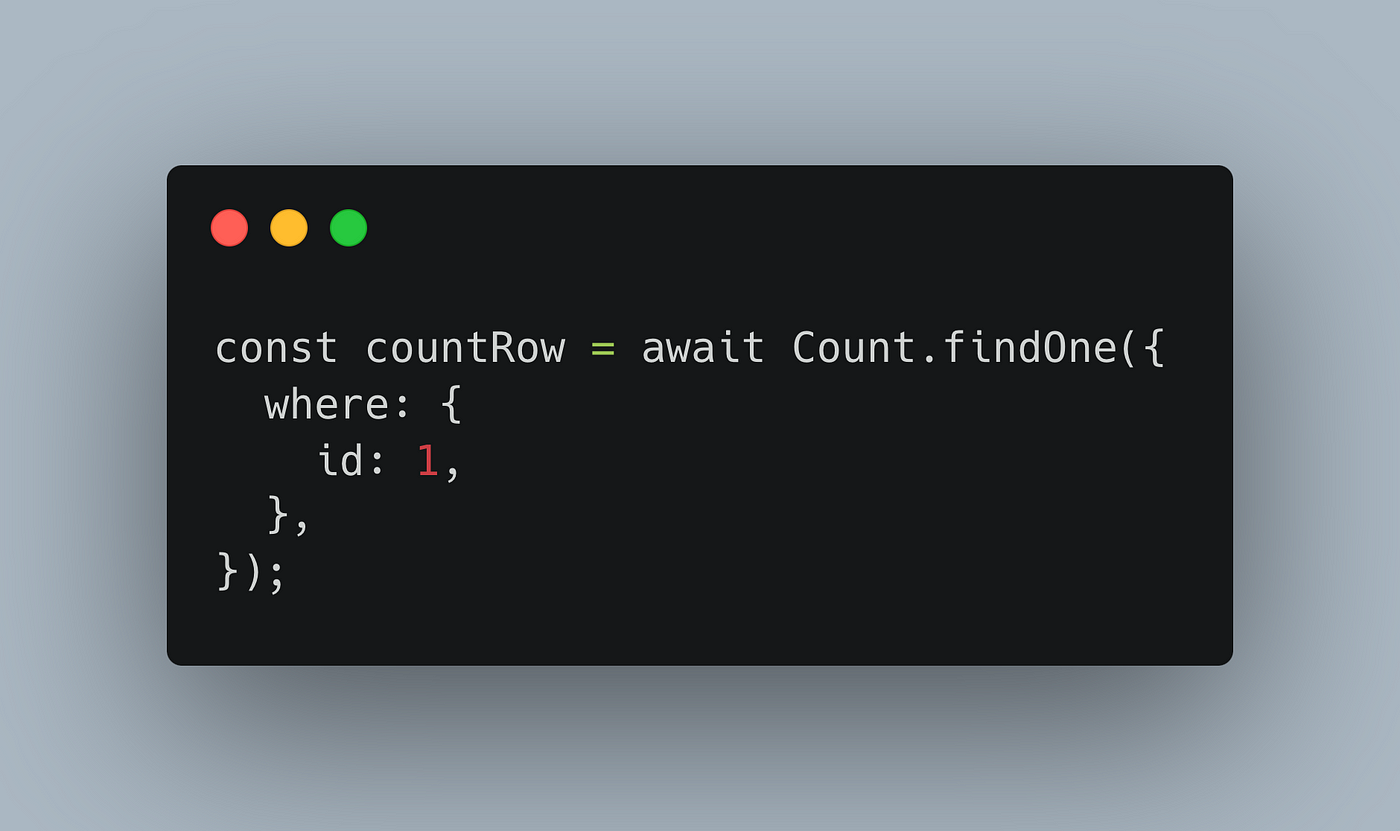
- At this point, both requests fetch the current count value, let’s say it’s initially 5.
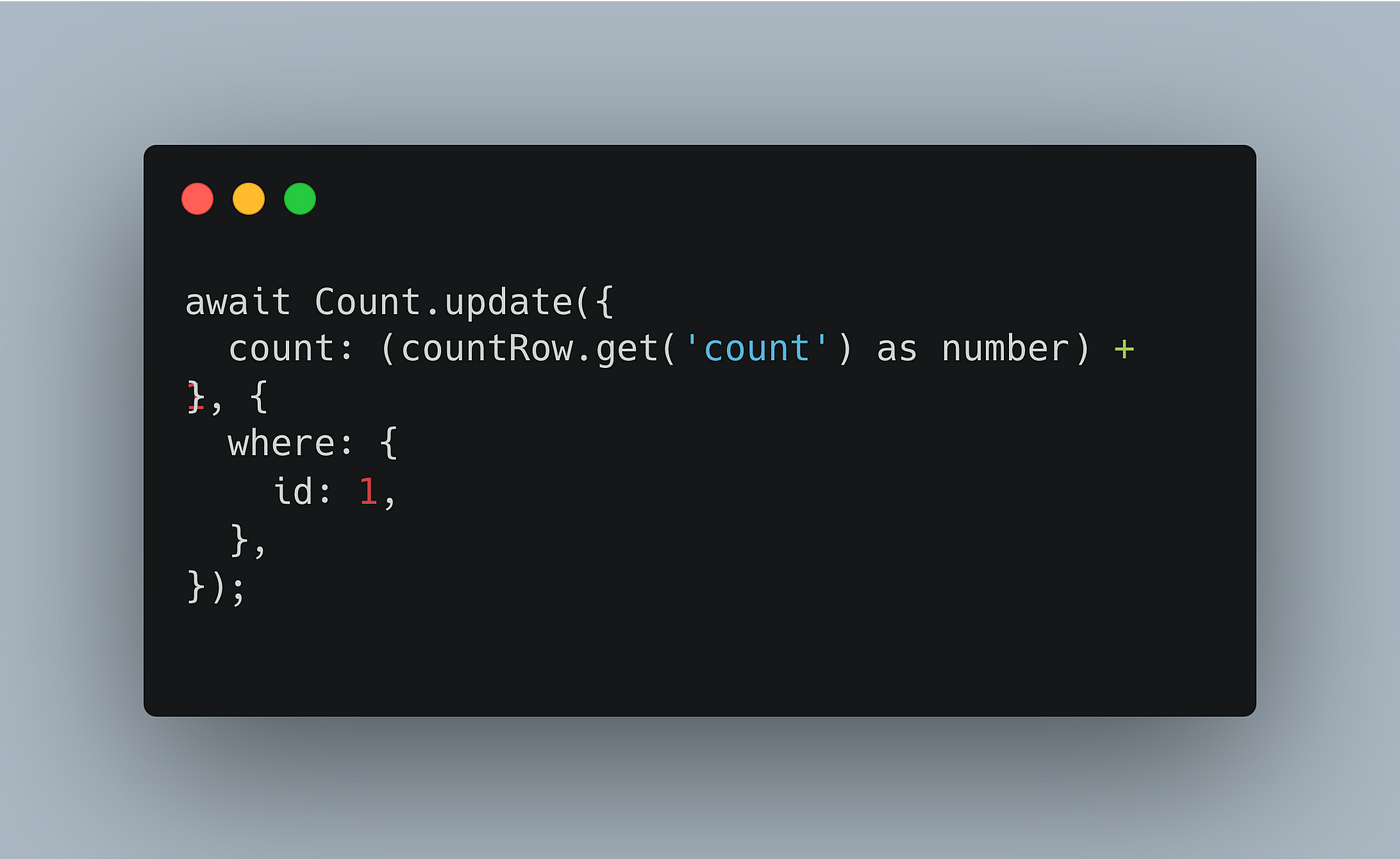
- Assuming the initial count is 5, both requests now want to update the count to 6.
Increment Operation
- Both requests increment the count value and attempt to update the database.
Database Update
- Request 1 updates the count to 6 in the database.
- Request 2, unaware of the change made by Request 1, also updates the count to 6.
Result
- The final count in the database is 6, even though both requests tried to increment it.
- One of the increments is effectively lost or overwritten by the other, leading to incorrect results.
This is a classic example of a race condition in concurrent programming. The issue arises because the two requests are not synchronized properly, and there’s no mechanism in place to ensure that the read and update operations happen atomically.
Without proper concurrency control mechanisms, multiple users can interfere with each other, leading to data inconsistencies and incorrect results.
Issue #1 —
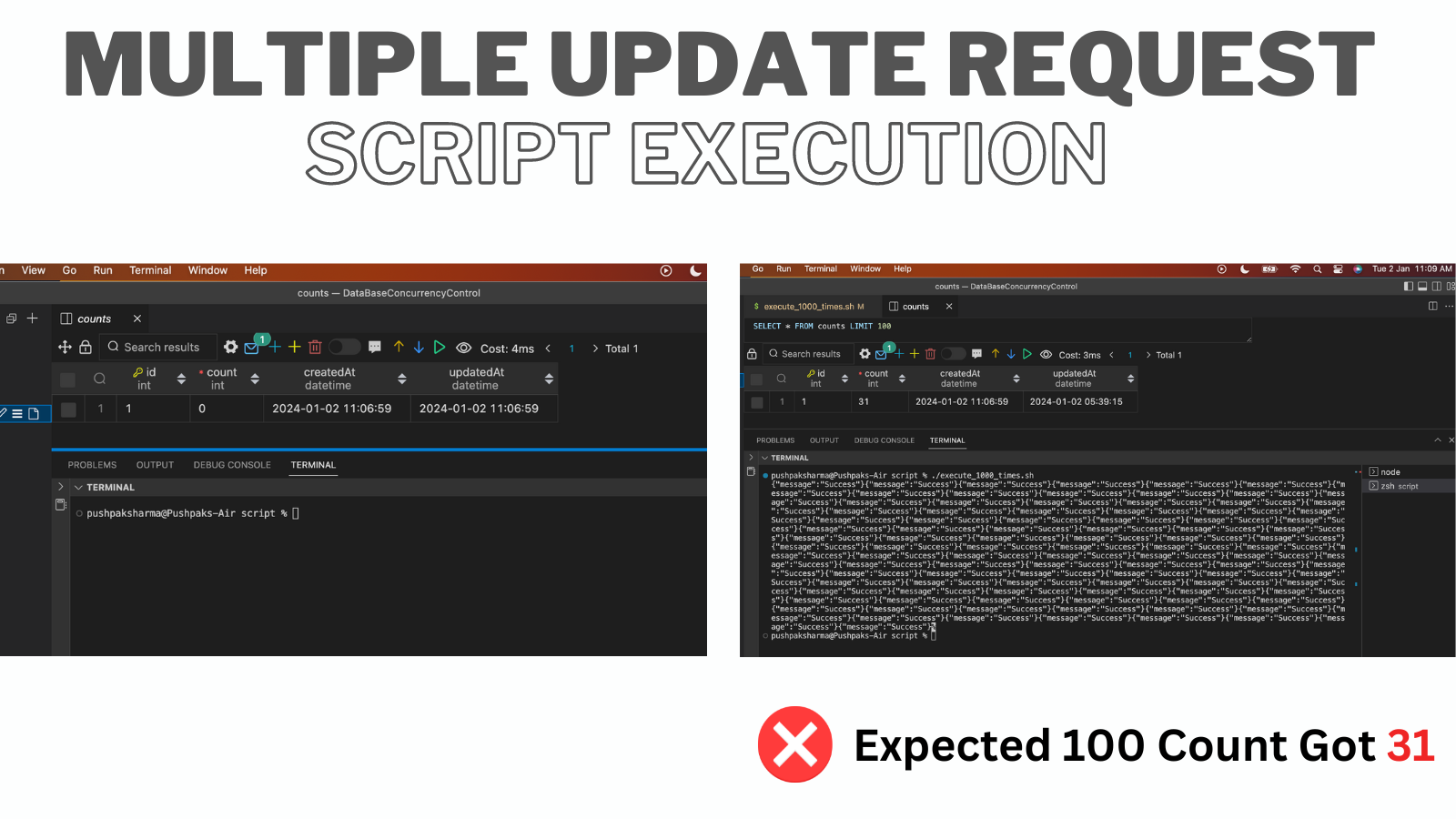
Approach
Implement Sequelize.Transaction for Database Concurrency Control
GitHub Issue:-
https://github.com/pushoo-sharma/Database-Concurrency-Control/issues/1
Transactions provide a way to manage concurrency and ensure the consistency of the database by allowing a series of database operations to be treated as a single, atomic unit.
By incorporating transactions, you make sure that the read and update operations are treated as a single, indivisible unit, preventing other transactions from interfering and maintaining data consistency. If an issue occurs during the transaction, it is rolled back, ensuring that the database remains in a valid state.
Issue #2 — Preventing Lost Update
Approach
Add Support for t.LOCK.UPDATE in Sequelize Transactions
GitHub Issue:-
https://github.com/pushoo-sharma/Database-Concurrency-Control/issues/2
Optimistic locking helps in scenarios where multiple transactions may attempt to modify the same record simultaneously. The primary reasons for using optimistic locking with t.LOCK.UPDATE
in Sequelize transactions are:
Preventing Lost Updates:
- Optimistic locking helps prevent the “lost update” problem. This occurs when two transactions read the same record, both make changes and then one transaction saves its changes, potentially overwriting the changes made by the other transaction.
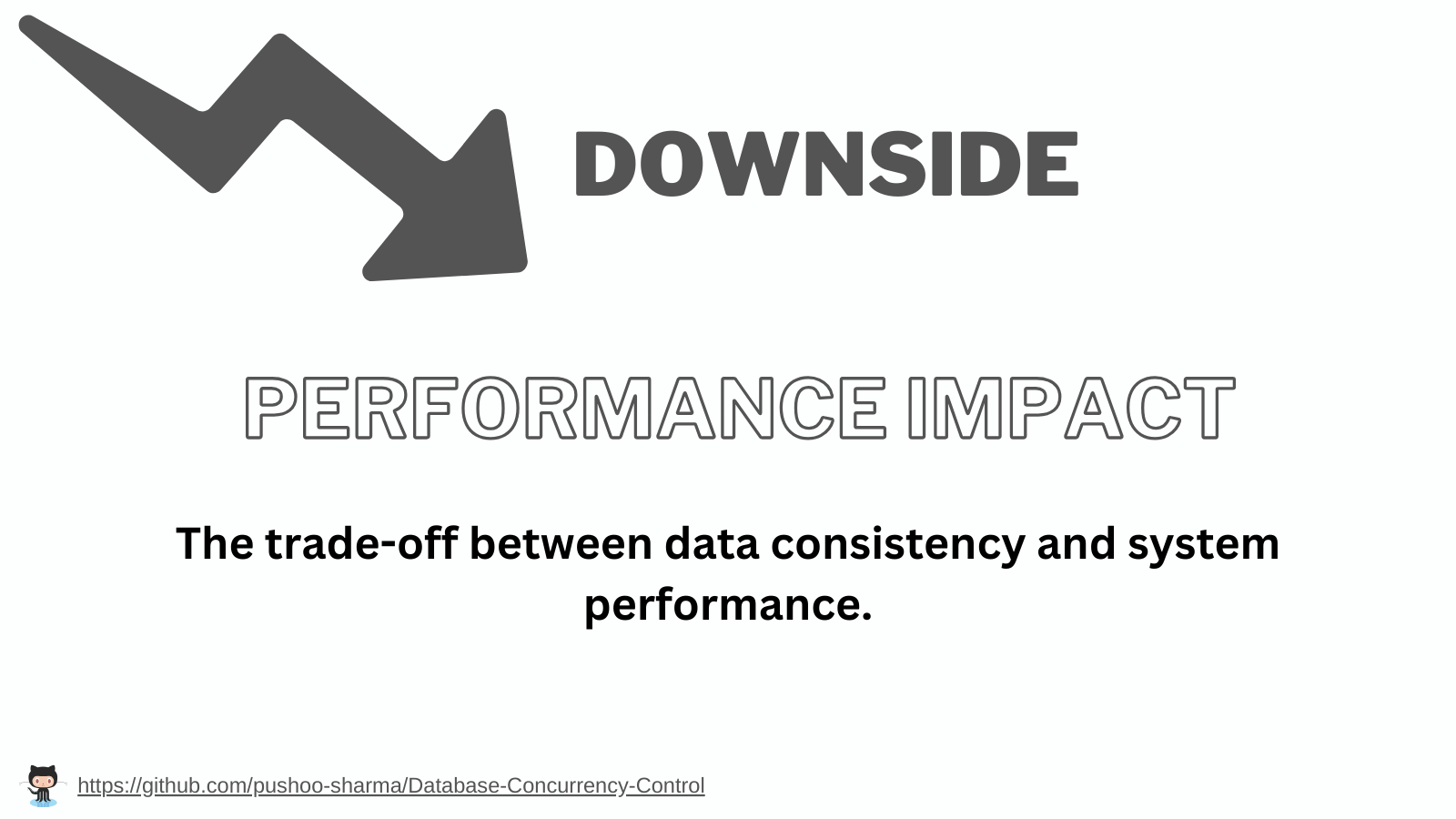
Issue #3 — Versioning Better performance
Approach
Add Versioning to Sequelize Model for Optimistic Concurrency Control
GitHub Issue:-
https://github.com/pushoo-sharma/Database-Concurrency-Control/issues/3
Versioning is a fundamental aspect of optimistic locking strategies. Optimistic locking assumes that conflicts are rare, and transactions proceed independently. The version number acts as an optimistic indicator that updates will likely succeed but is verified before committing changes.
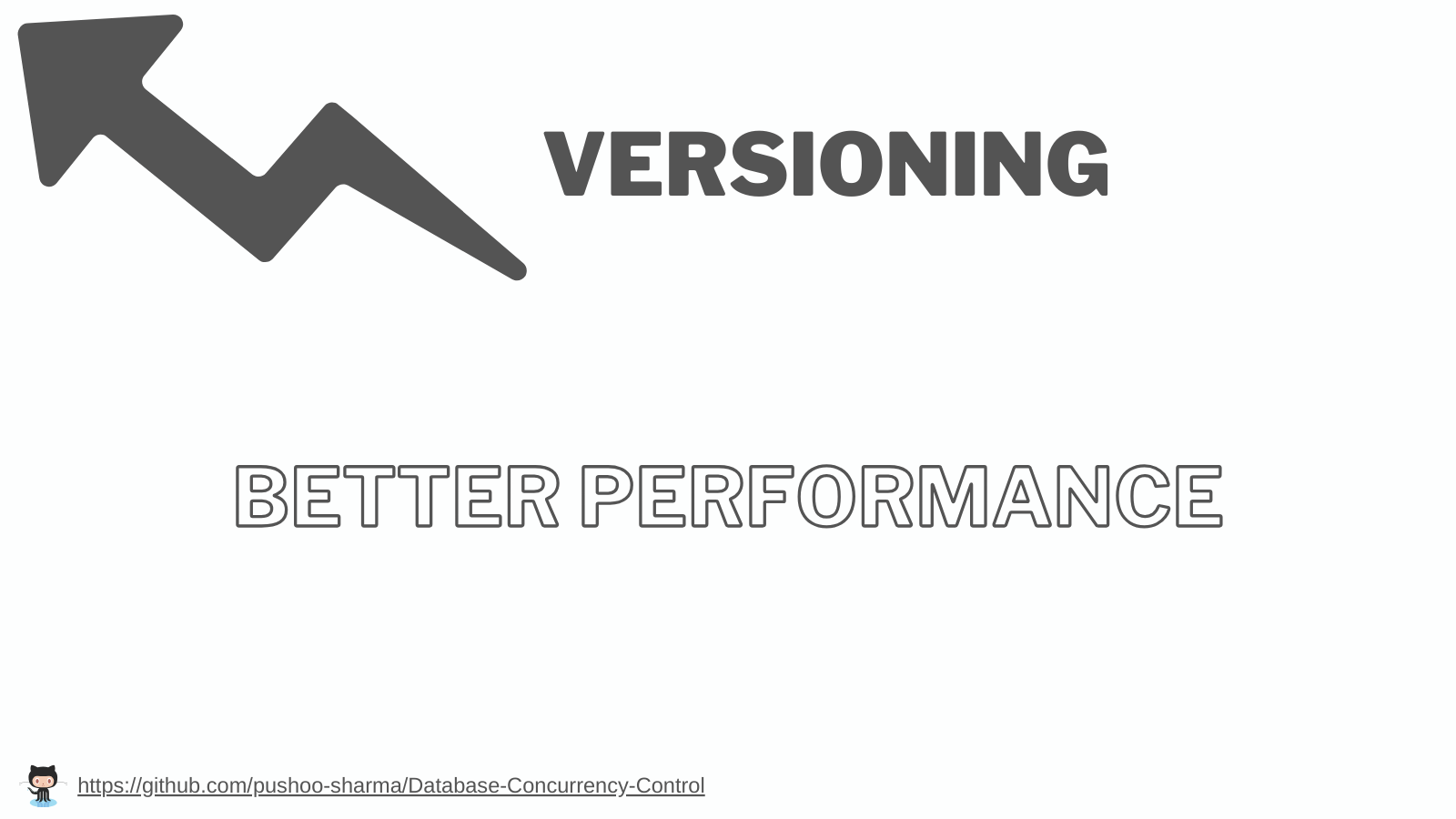
In summary, versioning is considered better in certain scenarios, especially when dealing with optimistic locking and concurrency control. It offers a balance between allowing concurrent transactions and ensuring data consistency by detecting and handling conflicts. The approach is particularly useful in situations where conflicts are infrequent, and the performance impact of locking entire records is a concern.
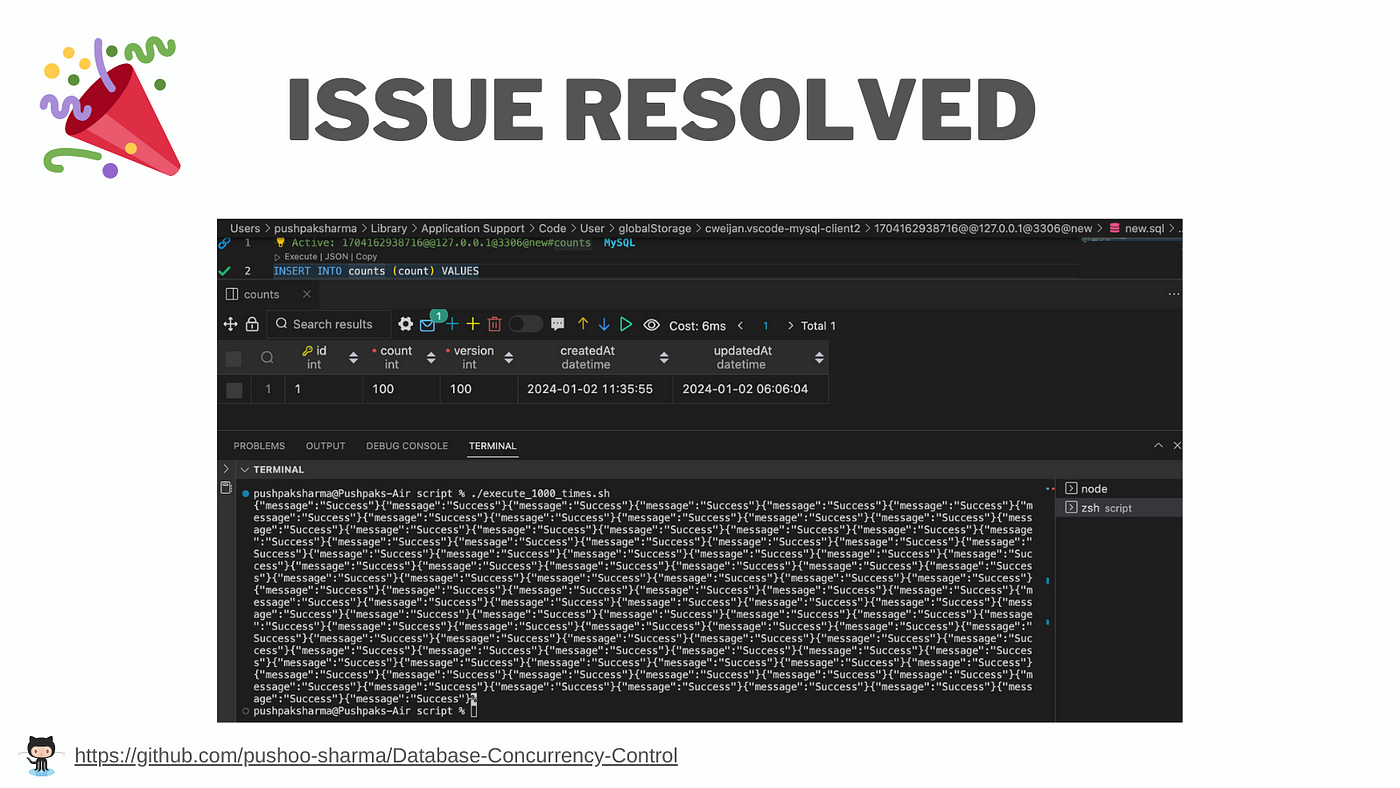
Summary and Question
Which database system, MySQL or DynamoDB, have you chosen to handle complex transactions, Consistency, and Transactions in your application?
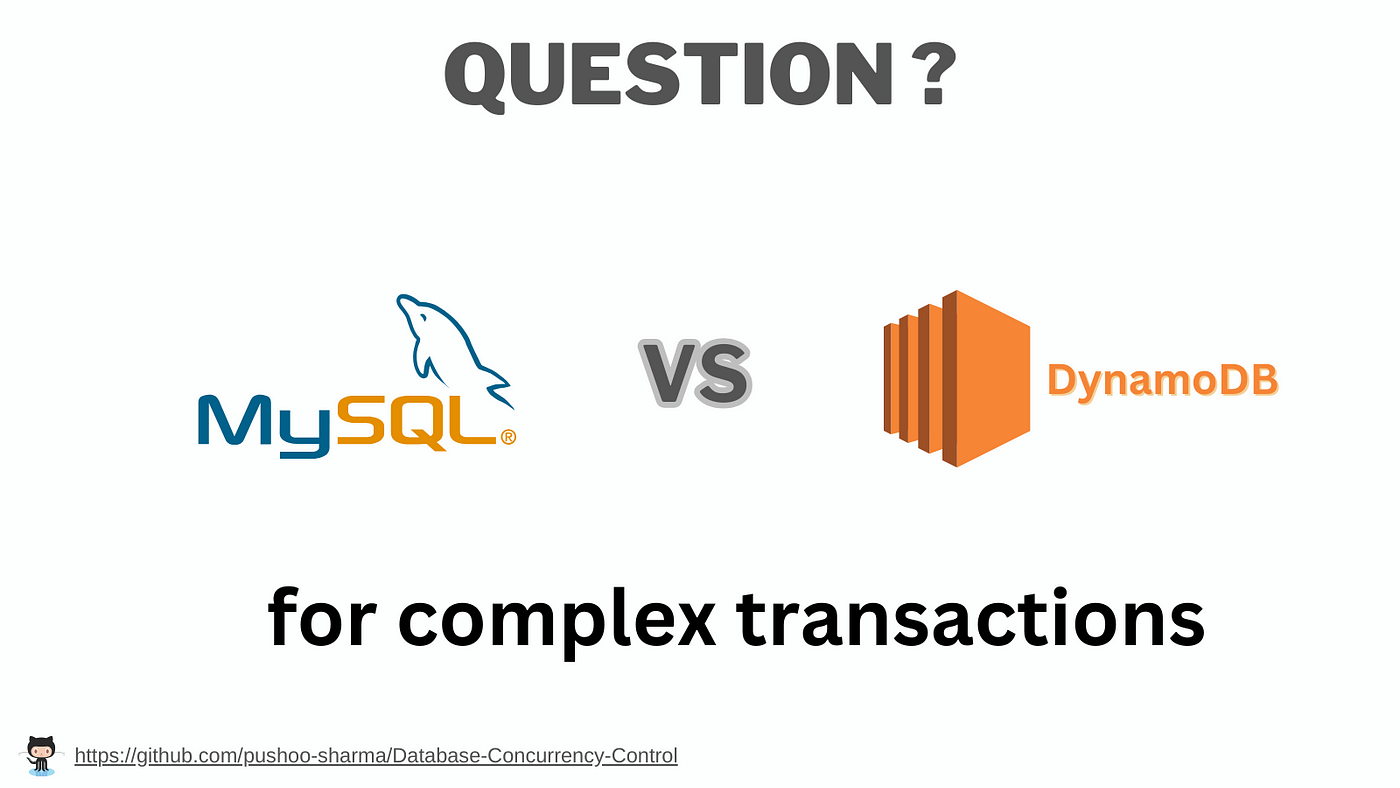